Getting started with PythonHere¶
Load the extension, and connect to the remote instance¶
%load_ext pythonhere
%connect-there
Execute some code on the remote¶
%%there
from kivy import platform
print("Hello from", platform)
Hello from android
Use Kivy widgets¶
%%there
from kivy.uix.label import Label
# Kivy's root widget is available via the `root` variable.
root.clear_widgets() # Remove all current childrens
# And add the new one
widget = Label(text="Kivy", font_size="50sp")
root.add_widget(widget)
%there screenshot -w 400
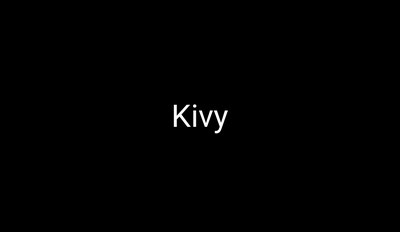
Objects introspection¶
%%there
print(root.children)
print(widget.color)
[<kivy.uix.label.Label object at 0x99d44db8>]
[1, 1, 1, 1]
Modify widget properties¶
%%there
widget.color = [1, .5, 0, 1]
widget.text += " Rocks!"
%there screenshot -w 400
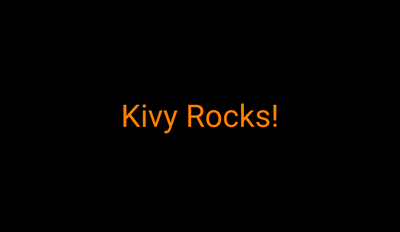
Make things dynamic with Clock¶
%%there
from kivy.clock import Clock
def clock_callback(delta_time):
widget.color[1] = (widget.color[1] + .1) % 1
clock = Clock.schedule_interval(clock_callback, 0.2)
%there -d .5 screenshot -w 400
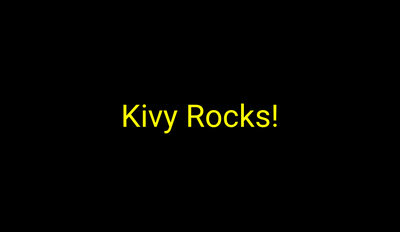
%%there
Clock.unschedule(clock)
Declare interface with the KV language¶
%%there kv
Button:
text: "Click me"
font_size: 10
on_release: self.font_size += 1
%there -d 5 screenshot -w 400
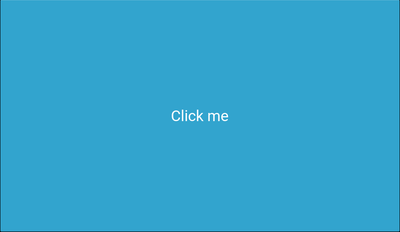
Combine Python with KV¶
%%there
from plyer import vibrator
from kivy.uix.button import Button
class VibroClone(Button):
def on_release(self):
self.root.add_widget(
VibroClone(root=self.root)
)
vibrator.vibrate(0.05 * int(self.text))
root.clear_widgets()
%%there kv
#:import get_random_color kivy.utils.get_random_color
#:import random random
<VibroClone>:
text: str(random.randint(1, 9))
color: get_random_color()
background_color: get_random_color()
size_hint: 1, 1
GridLayout:
cols: 10
size_hint: 1, 1
VibroClone:
root: root
%there -d 4 screenshot -w 400
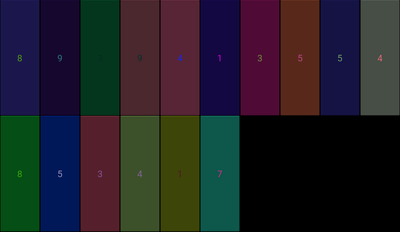